Water activated switch
I thought that it would be cool to create a switch, which is turned on by water contact. This means when the switch is closed, it indicates that the device - e.g. DiveIno - is in the water.
Wiring
I took one of my Arduino Mega boards and hooked up the following components with each other based on this layout:
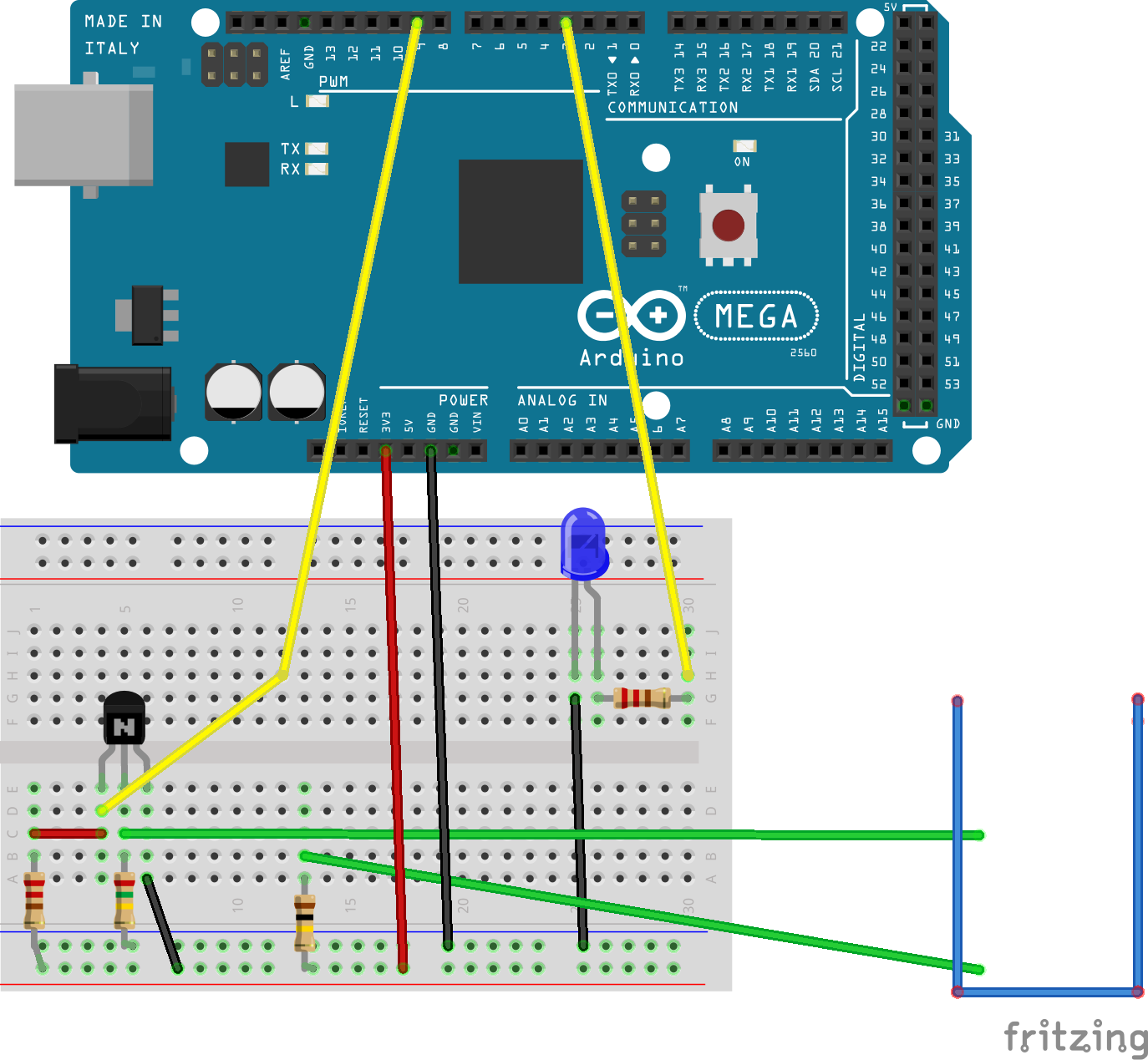
The two green wires should go into a water filled bowl depicted by a blue wire on the right hand side.
Parts
Based on this diagram you need the following parts:
- Arduino Mega microcontroller board
- BC547 NPN transistor
- Blue LED
- 250 kilo Ohm resistor
- 100 kilo ohm resistor
- 2x 220 Ohm resistor
- Breadboard wires
Sketch
In order to make the prototype circuit work I wrote the following Arduino sketch:
1 | int LED_PIN = 3; |
The LED is connected to the digital 3 pin, the transistor collector is to the digital 9 pin of the Arduino Mega board.
Water activated switch
If you put the two green wires into a water filled bowl then the WATER SWITCH - ON message will be printed to the Serial Monitor and the blue LED will turn on.
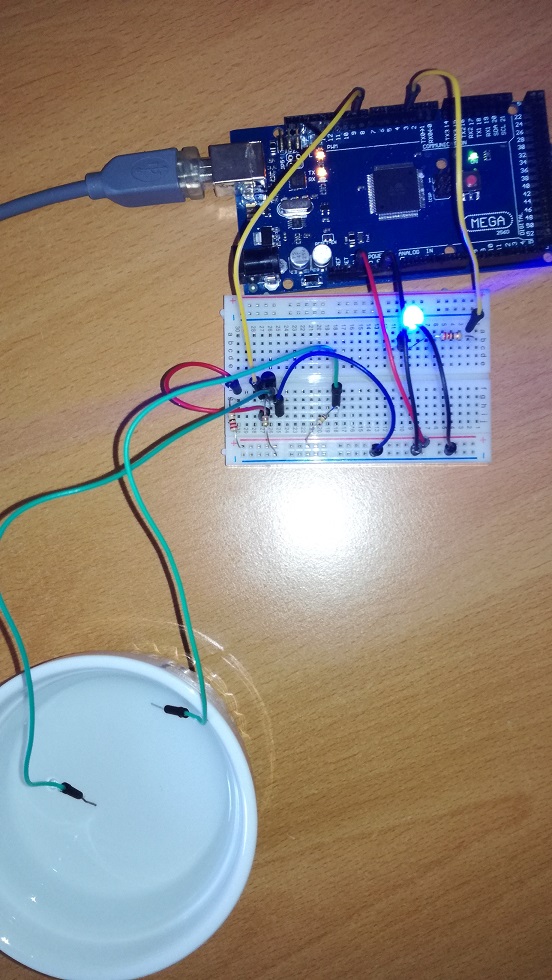
If one of the wires are removed from the water, the connection gets broken. In this case the WATER SWITCH - OFF message gets printed to the Serial Monitor and the LED is turned off.
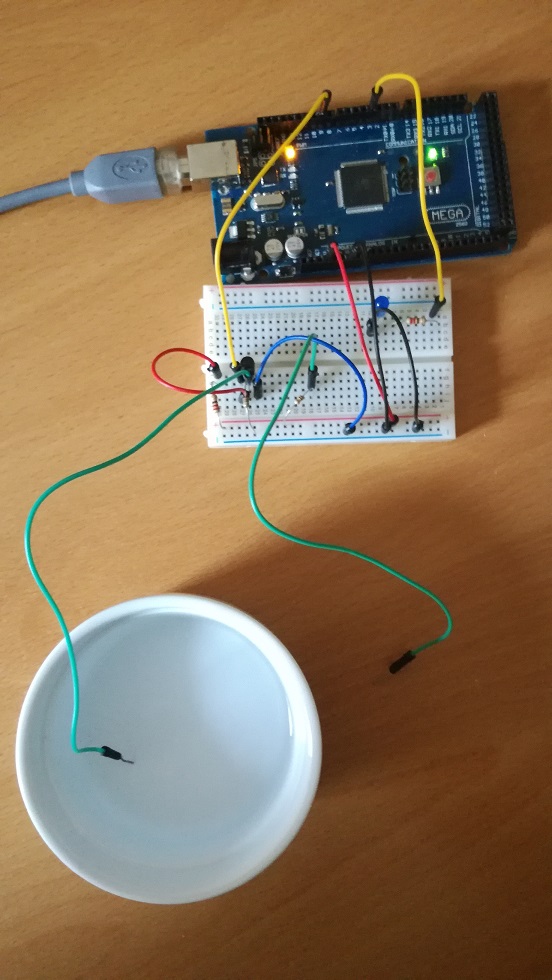
After some time - governed by the ACTIVATION_LEVEL value - the activatedWaterSwitch() method in the sketch gets called and it prints the WATER SWITCH - Activated message to the Serial Monitor.
Conclusion
I played a bit with this prototype circuit. I submerged the wires into sweet and salt water. Salt water is more conductive than sweet water, therefore the wire tips can be at the other side of the bowl, the connection is still established. However in sweet water the distance between the tips do matter! The closer is the better! If the tips are too far away from each other, there will be no connection at all.
If you would like to activite your device by water contact make sure that the wire tips be close to each other!
Water level measurement
Another option is to use this circuit as a basis to measure the water level in a tank. The Arduino Water Level Indicator blog post contains an excelent description about how this can be done.